TxTranslation
Simple yet powerful translation and localisation library for .NET applications. Supports XAML binding, language fallbacks, count-specific translations, placeholders, and number and time formatting.
It only takes about 4 minutes to read this page.
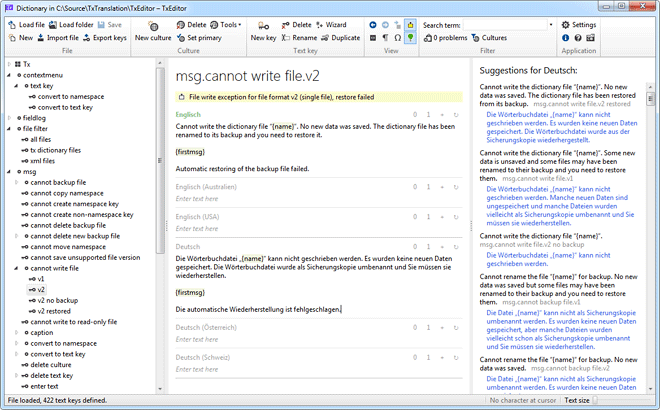
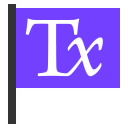
tl;dr: Overview of the advantages and features:
The Tx concept
Tx is a simple yet powerful translation and localisation library for .NET applications. It manages a dictionary containing all the text snippets and phrases you need, for multiple translations. If a translation is not available in your preferred language, it can be looked up from other languages. Texts can also contain named place-holders that are filled with your data at runtime so that you don’t need to concatenate all the parts yourself in the right order. Localisation tasks like typography, number or time formatting according to the local standards are also covered.
A special feature of Tx dictionaries is that each text can have different translations depending on the subject count you’re talking about, also called pluralisation. One day is a different word than two days. This allows you to speak to your users in the most natural way, avoiding ugly parentheses or alternatives for plural words in the user interface.
All texts and translations are stored in an XML dictionary file, one per project. This XML file usually contains all languages. The dictionary can be installed with your application, or compiled right into it as an embedded resource.
The TxLib library can be used from any .NET application and is the part you’ll distribute with your application. For more portable applications, you could also directly copy the class files into your project – it’s only a few files. Its main class, Tx
, is further described in the documentation.
Text keys
All texts are associated with text keys. A text key is a short string that uniquely identifies a text or phrase and is usually used in the application source code to refer to a specific text. Text keys can be freely assigned by the developer, but there are some structuring guidelines described at the end of the documentation and a few additional requirements when using the TxEditor tool.
Cultures
The .NET framework uses the concept of cultures to specify a combination of language and data formatting rules. Each culture has a code that describes the language and optionally a region. There are more variants but they are not supported by TxLib. Examples are “en” for the English language, “de-DE” for the German language in Germany or “pt-BR” for the Portuguese language in Brasil (as opposed to Portugal which many would probably first think of). TxLib uses these cultures to identify translations and both, region-specific and language-only codes, are used where the latter ones always serve as fallback if a translation is not found for a specific culture.
TxEditor
The TxTranslation solution also comes with a graphical translation file editor that provides all the functionality for translating personnel that don’t necessarily have any programming experience. The TxEditor application can load a dictionary file, list all the text keys that it defines and allows viewing and editing the translation texts. It performs consistency checks to point the user to potential errors and highlight missing translations. TxEditor also makes use of TxLib itself so it is fully localisable and can be used to translate itself into other languages.
Compatibility: Version 4.0 or newer
Example
The following C# example shows several usage possibilities of the Tx class.
Tx.T("my text key")
// Count-specific translations:
Tx.T("days", daysCount)
// Use of named placeholders:
Tx.T("user info", // The text key
"name", userName, // Pairs of placeholder names and values
"location", User.Location,
"status", statusValue)
// Quote text (put it in local quotation marks):
Tx.Quote(someText)
// Combine translation and quote:
Tx.QT("my text key")
// Date formatting:
Tx.Time(DateTime.Now, TxTime.MonthDayLong)
// Relative time formatting:
Tx.RelativeTime(someTime)
// Bonus: ISO 8601 time interval handling:
new DateTimeInterval(DateTime.Now, "PT3H25M").TimeSpan
// Dictionary culture listing (returns string[]):
Tx.AvailableCultureNames
The following XAML example shows several usage possibilities of the WPF bindings.
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:Tx="http://unclassified.software/source/txtranslation" ...>
<StackPanel>
<TextBlock Text="{Tx:T my text key}"/>
<TextBlock Text="{Tx:T n months, {Binding Value, ElementName=Counter}}"/>
<TextBlock Text="{Tx:Number {Binding Power}, 3, Unit=kW}"/>
<TextBlock Text="{Tx:DataSize {Binding FileSize}}"/>
<TextBlock Text="{Tx:Time {Binding LastUpdate}, Details='DowLong,YearMonthDay'}"/>
</StackPanel>
The documentation (docx and pdf in the code repository) describes the TxLib methods usage as well as the TxEditor application more detailed.
Images
Here are several screenshots of the TxEditor application:
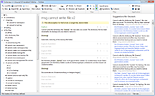

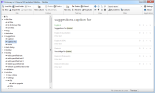

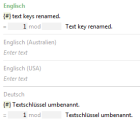

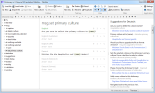

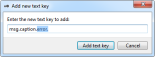

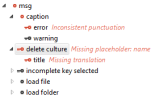

Download
Note: Releases after 2016-01-09 are no longer digitally signed because there are no more free code signing certificates available for open source developers. A commercial certificate from 200 € per year doesn’t pay for me. I’m sorry for the confusion this may cause with your next download.
TxChanges.txt2.3 KiBChange log of published versions
master.zipLatest source code directly from GitHub
TxSetup-1.383.76_da9f9b.exe1.7 MiBVersion 1.383.76 of 2016-01-19, Commit da9f9b
TxSetup-1.184.37_7f3858.exe1.7 MiBVersion 1.184.37 of 2015-07-04, Commit 7f3858
TxSetup-1.113.79_b4ffb0.exe1.7 MiBVersion 1.113.79 of 2015-04-24, Commit b4ffb0
TxSetup-1.53.78_a21990.exe1.7 MiBVersion 1.53.78 of 2015-02-23, Commit a21990
TxSetup-2fjy.0ee169.exe1.7 MiBVersion 2fjy of 2015-01-28, Commit 0ee169
TxSetup-26w6.35374c.exe1.7 MiBVersion 26w6 of 2014-11-16, Commit 35374c
TxSetup-21pr.407c11.exe1.7 MiBVersion 21pr of 2014-09-21, Commit 407c11
TxSetup-207t.4c2590.exe1.6 MiBVersion 207t of 2014-09-05, Commit 4c2590
You can also head over to GitHub to download any previous revision or check the issue tracker:
TxTranslation repository on GitHub
This package is also available through NuGet. That way, it can be integrated and updated in a Visual Studio project very quickly. This package only includes the TxLib assembly for .NET 4.0. For installing TxEditor you need to download the setup program above.
Unclassified.TxLib package on NuGet
Current development state
TxLib and the dictionary file format are now considered stable but little tested yet. There are plans for future additional features but no API change.
TxEditor is still new and also incomplete. Some indicated functions are missing or stop working in some conditions. The user interface is not yet rock-solid or even very clean. This is currently being improved.
The documentation is almost complete, but some sections about unfinished parts in TxEditor are also missing. The source code reference is not ready for the public yet, use the inline comments through IntelliSense instead (original source code or .xml file).
Changes
- TxEditor: Text key wizard: Preset language from current file type in the Visual Studio editor
- TxEditor: Text key wizard: ASP.NET Razor .cshtml files are handled for code and content text
- TxEditor: Text key wizard: "Add colon" option when one was found in the text
- TxEditor: Text key wizard: Skip unused placeholders in code generation
- TxEditor: Text key wizard: Enter key in "Similar texts" list select key instead of clicking the OK button
- TxEditor: Text key wizard: Fixed file extension recognition for unsaved files (* after file name)
- TxEditor: Text key wizard: Fixed parsing end of string quote within one level of parentheses in code
- TxEditor: Load files selection: Double click opens file when the list is complete
- TxEditor: Load files selection: Automatically load only found file with /scan option
- TxEditor: Consider ellipsis character for inconsistent punctuation
- TxEditor: Only accept numeric values in count and module fields
- TxEditor: Avoid flickering of main window after closing text key wizard dialog
- TxEditor: Writing XML comment with TxEditor download link into .txd files
- Updated documentation (docx only)
- TxEditor: Copy text key to clipboard from the context menu
- TxEditor: Text key wizard: Support for C# 6 interpolated strings
- TxEditor: Text key wizard: C# 6 string parsing, accelerator keys
- TxLib: Obfuscation compatibility for XAML use
- Shared code update and cleanup, updated formatting to new style guide
- Update to Visual Studio 2015
- TxLib: Fix: .txd file is not reloaded when saved with TxEditor
- Allow disabling special verbose single-unit texts in Tx.TimeSpanRaw for generic use
- Build script and tools updated
First log entry in this file.
For a full list of changes please refer to the Git repository commit history.
Licence and terms of use
The TxLib library, which is used in applications, is released under the terms of the GNU LGPL licence, version 3. The other projects (TxEditor, demos and converters) are released under the terms of the GNU GPL licence, version 3. You can find the detailed terms and conditions in the download or on the GNU website (LGPL, GPL).
Statistic data
- Created on 2013-07-01, updated on 2016-01-26.
- Ca. 15 300 lines of code, estimated development costs: 15 000 - 61 000 €